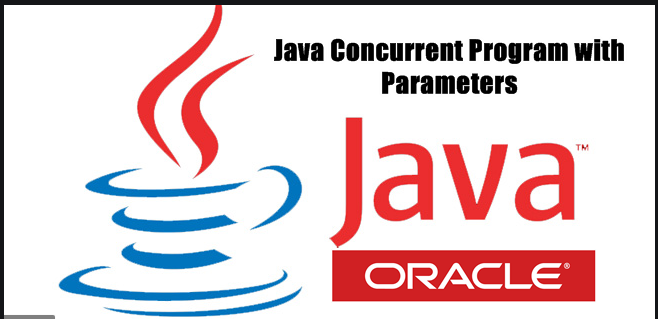
Java Concurrent Program with Parameters in Oracle Apps R12
We have seen how to create Java concurrent program in Oracle Apps R12.
In this article, we will see how to Java concurrent program with Parameters and how to some of the global apps columns/fields like RequestId, UserId and etc.
While submitting Java concurrent program we will pass some parameters and those parameters we will retrieve in the Java program.
Create Java Concurrent Program with Parameters
Let’s create a concurrent program with 3 different parameters.
Note: we use the same java program and concurrent program that we used in another post.
Java Program
Now let’s try to retrieve those parameter values inside the Java program.
The above code gets all the pramaters list from the conccurrent program. In our case all the 3 parameters.
Since we have 3 values we have to use the looping technique.
All 3 parameters with different data types but while retrieving in Java all those are character data types.
Integer.parseInt(para.getValue()) — So we have to convert the String to Number
(Date)dateFormat.parse(para.getValue()) — Convert String to Date
If we pass the date parameter via a concurrent program we have to the Oracle default format type is yyyy/MM/dd HH:mm:ss.
So while receiving the date data type we have to convert the same format and use it in our program.
SimpleDateFormat dateFormat = new SimpleDateFormat(“yyyy/MM/dd HH:mm:ss”);
Print the retrieved parameters in the Outfile of the concurrent program.
To get some of the Global columns like RequestID, UserId, UserName, ResponsibilityId and etc.. Use the below code.
To make concurrent program Normal completion/Warning/Error.
package cus.oracle.apps.cus.javaconc; import java.text.SimpleDateFormat; import java.util.Date; import oracle.apps.fnd.cp.request.CpContext; import oracle.apps.fnd.cp.request.JavaConcurrentProgram; import oracle.apps.fnd.cp.request.LogFile; import oracle.apps.fnd.cp.request.OutFile; import oracle.apps.fnd.cp.request.ReqCompletion; import oracle.apps.fnd.cp.request.ReqDetails; import oracle.apps.fnd.util.NameValueType; import oracle.apps.fnd.util.ParameterList; public class JavaConcurrentProg implements JavaConcurrentProgram{ public JavaConcurrentProg() { } OutFile out; LogFile log; public void runProgram(CpContext cpContext) { out = cpContext.getOutFile(); log = cpContext.getLogFile(); int Parameter1 = 0; String Parameter2 = ""; Date Parameter3 = new Date(); //SimpleDateFormat dateFormat = new SimpleDateFormat("dd-MMM-yyyy"); SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss"); log.writeln("This is the first program", 0); out.writeln("This is the first Java Concurrent Program"); // Getting Parameters ParameterList parameters = cpContext.getParameterList(); try{ //Loop the parameters list to get the parameters one by one while(parameters.hasMoreElements()){ NameValueType para = parameters.nextParameter(); if ("Number".equals(para.getName())){ Parameter1 = Integer.parseInt(para.getValue()); } else if("Char".equals(para.getName())){ Parameter2 = para.getValue(); } else if("date".equals(para.getName())){ // Parameter3 = para.getValue(); Parameter3 = (Date)dateFormat.parse(para.getValue()); } } } catch(Exception e){ out.writeln("Exception at Parameters reading "+ e.getMessage()); } out.writeln("Parameter 1: "+Parameter1); out.writeln("Parameter 2: "+Parameter2); out.writeln("Parameter 3: "+Parameter3); ReqDetails reqDetails = cpContext.getReqDetails(); out.writeln("Request Id: "+ reqDetails.getRequestId()); out.writeln("User Id: "+ cpContext.getUserId()); out.writeln("User Name: "+ cpContext.getUserName()); out.writeln("Responsibility Id: "+ cpContext.getRespId()); cpContext.getReqCompletion().setCompletion(ReqCompletion.NORMAL, "Completed."); // To make concurrent program Normal //cpContext.getReqCompletion().setCompletion(ReqCompletion.ERROR, "Completed."); -- To make concurrent program Error //cpContext.getReqCompletion().setCompletion(ReqCompletion.WARNING, "Completed."); -- To make concurrent program Warning } }
Migrate the java file to the Oracle server custom path and generate the class file using the below command.
Using WinSCP tool we can migrate the above java program into $JAVA_TOP/cus/oracle/apps/cus/javaconc path.
javac -cp .:/u01/data_app/apps/apps_st/comn/java/classes JavaConcurrentProg.java
Run the concurrent program and see the output