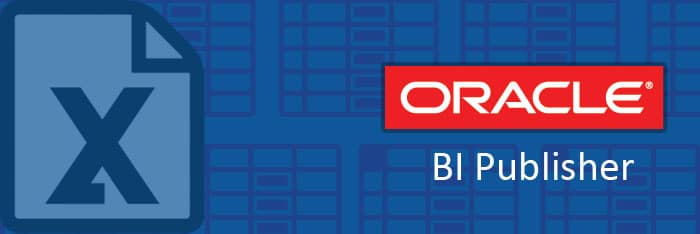
Submitting XML Concurrent Program from Backend(PLSQL)
Here we talk about how to submit the XML based concurrent program from backend using PLSQL.
In order to submit the XML concurrent program in R12 and above, we have to follow the below steps.
1. Assign an operating unit value(optional)
2. Initialize the session variables
3. Add the XML template using Oracle standard API
4. Submit the concurrent program using Oracle standard API
Assign an operating unit value: Sometimes in Oracle Apps R12 onwards, if we enable to multi-org feature then we have to select the Operating unit value while submitting the concurrent program.
We have to use below API to set the OU value for the concurrent program.
fnd_request.set_org_id(92);
92 is the operating unit id, we can find this value from HR_OPERATING_UNITS table.
Initialize the session variables: We have to set up a user id, application id, and responsibility id.
If we want to see the concurrent program out file and log file based on a responsibility that you have access, then provide that responsibility id and application id(application short name) and user id values.
fnd_global.apps_initialize (l_user_id,l_responsibility_id,l_application_id);
To get responsibility id, application id and user id use below SQL’s.
BEGIN SELECT DISTINCT fr.responsibility_id, fr.application_id INTO l_responsibility_id, l_application_id FROM apps.fnd_responsibility fr, apps.fnd_responsibility_tl frt WHERE fr.responsibility_id = frt.responsibility_id AND UPPER (frt.responsibility_name) = UPPER ('Receivables Manager'); EXCEPTION WHEN OTHERS THEN l_responsibility_id := NULL; l_application_id := NULL; END;
BEGIN select user_id into l_user_id from apps.fnd_user where user_name like 'USER'; EXCEPTION WHEN OTHERS THEN l_user_id := NULL; END;
Add the XML template using Oracle standard API
Before submitting the concurrent program we need to assign an XML layout(Template) to the concurrent program.
If we look at the SRS window in Oracle EBS we could notice layout name while we are submitting the XML based concurrent program.
We can achieve the same functionality in PLSQL by below Oracle standard API.
fnd_request.add_layout
Submit the concurrent program using Oracle standard API
After all the above three steps, we can submit the concurrent program using fnd_request.submit_request.
-- Submit the XML concurrent program from back end(using PLSQL) DECLARE l_layout BOOLEAN; l_request_id NUMBER; l_responsibility_id NUMBER; l_application_id NUMBER; l_user_id NUMBER; BEGIN BEGIN SELECT DISTINCT fr.responsibility_id, fr.application_id INTO l_responsibility_id, l_application_id FROM apps.fnd_responsibility fr, apps.fnd_responsibility_tl frt WHERE fr.responsibility_id = frt.responsibility_id AND UPPER (frt.responsibility_name) = UPPER ('Receivables Manager'); EXCEPTION WHEN OTHERS THEN l_responsibility_id := NULL; l_application_id := NULL; END; BEGIN select user_id into l_user_id from apps.fnd_user where user_name like 'USER'; EXCEPTION WHEN OTHERS THEN l_user_id := NULL; END; -- TO set the concurrent program operating unit value fnd_request.set_org_id(92); -- Initialize the fnd variables fnd_global.apps_initialize (l_user_id,l_responsibility_id,l_application_id); dbms_output.put_line(l_user_id||'-'||l_responsibility_id||'-'||l_application_id); -- Add the layout /* you can get the layout/template details using below tables xdo_templates_b xdo_templates_tl you can pass the template_code as concurrent program short name */ l_layout := fnd_request.add_layout( template_appl_name => 'GL' -- Application Short Name ,template_code => 'XYZ' -- XML template code ,template_language => 'en' ,template_territory => 'US' ,output_format => 'PDF' ); -- Submit the concurrent program l_request_id := fnd_request.submit_request ( application => 'GL' -- Application Short Name ,program => 'XYZ' -- Concurrent program short name ,description => 'Concurrent Program Description' ,start_time => SYSDATE -- Concurrent program run date ,sub_request => FALSE ,argument1 => 'NUMBER' -- Arguments1..n are the concurrent program parameters ,argument2 => NULL ,argument3 => NULL ,argument4 => 123456 ,argument5 => 123456 ,argument6 => NULL ,argument7 => NULL ,argument8 => NULL ,argument9 => NULL ,argument10 => NULL ); COMMIT; IF l_request_id = 0 THEN dbms_output.put_line ('Unable to submit the concurrent program'); ELSE dbms_output.put_line('Successfully Submitted the Concurrent Program and Request Id: '||to_char(l_request_id)); END IF; EXCEPTION WHEN OTHERS THEN dbms_output.put_line('Error in the process '||SQLCODE||SQLERRM); END;